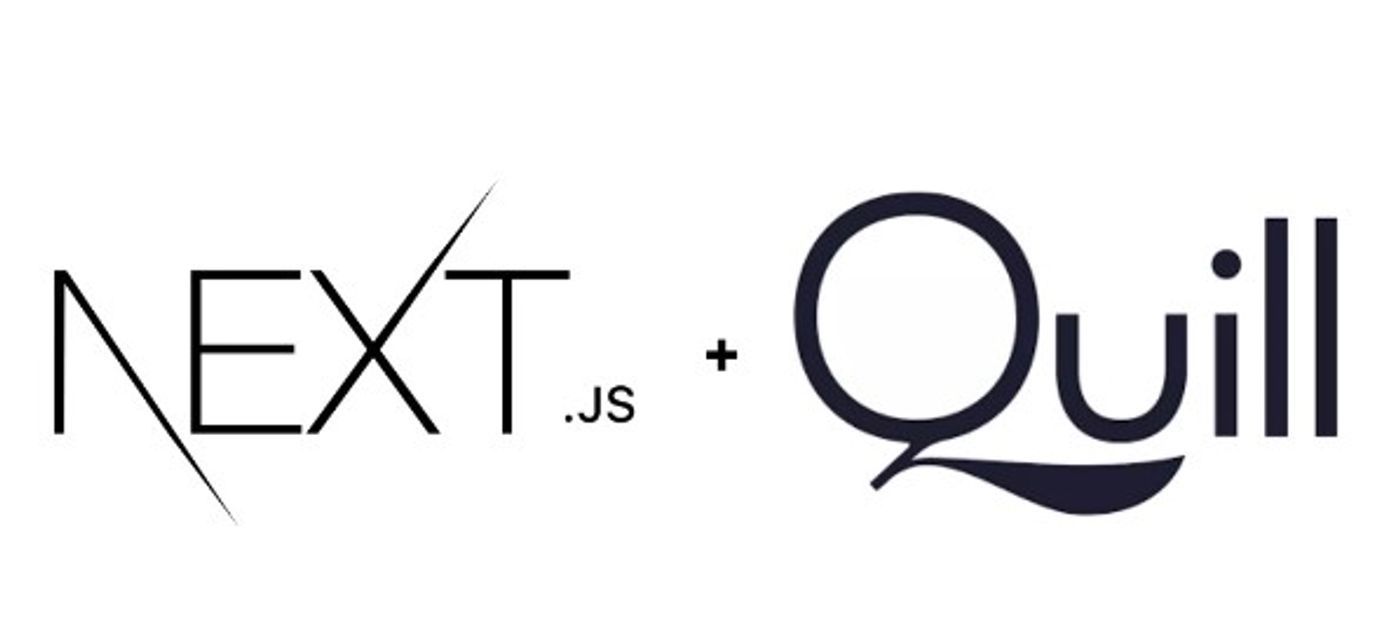
SHIFT + ENTER in React Quill to create a new line 100% working

Table Of Contents
React Quill is a popular WYSIWYG (What You See Is What You Get) text editor for React applications. It provides a rich text editing experience with features like formatting, bullet points, and more. However, one common feature that may not be available out of the box is the ability to create a new line when pressing SHIFT + ENTER. In this blog post, we'll walk you through the steps to add this functionality to React Quill.
Before diving into the code, ensure React Quill is installed in your project. If not, you can install it using npm or yarn:
npm install react-quill # or yarn add react-quill
Step 1: Import React Quill
In your React component, import React Quill:
// = = = = =
// IMPORT REACT QUILL
// = = = = =
const ReactQuill = dynamic(
async () => {
const { default: RQ } = await import("react-quill");
RQ.Quill.register("modules/handleImageUpload", RQ);
return function forwardRef({ forwardedRef, ...props }: { forwardedRef: React.Ref<any>; [key: string]: any }) {
return <RQ ref={forwardedRef} {...props} />;
};
},
{
ssr: false,
loading: () => <p>Loading ...</p>,
}
);
Step 2: Customize the Enter Key Behavior
To customize the Enter key behavior, you'll need to modify the Quill editor's keyboard bindings. Specifically, you'll disable the default Enter behavior and add a custom binding for Shift + Enter to insert a line break. Here's how to do it:
const PostEditIndex = () => {
// REACT QUILL STEP 2:
const quillRef = useRef<any>(null);
const modules = useMemo(
() => ({
keyboard: {
bindings: {
// Disable the default Enter behavior (inserts a new paragraph)
enter: false,
// Define a custom binding for "Shift + Enter" to insert a line break
customShiftEnter: {
key: 13, // Enter key code
shiftKey: true,
handler: () => {
const quill = quillRef.current.getEditor();
const keyboard = quill.getModule("keyboard");
delete keyboard.bindings[13];
const range = quill.getSelection();
let position = range ? range.index : 0;
quill.insertText(position, "\n", "break"); // create a line break with p tag
return;
},
},
},
},
toolbar: {
container: [
// YOUR REST CONTAINER
["break"],
],
},
clipboard: {
matchVisual: false,
},
}),
[]
);
const formats = ["header", "font", "size", "bold", "italic", "underline", "break"];
In this code:
- We define a function customShiftEnter to customize the Enter key behavior.
- Inside this function, we access the Quill instance and its keyboard module.
- We disable the default Enter behavior (new paragraph) by setting keyboard.bindings.enter to false.
- We add a custom binding for Shift + Enter to insert a line break (<br>).
- The default Enter binding (key code 13) is deleted to prevent any conflicts.
- Finally, we insert the line break and move the cursor to the appropriate position.
Step 3: Using the Customized Editor
You can now use the ReactQuill component in your application, and it will enable Shift + Enter to insert line breaks as desired:
<ReactQuill
defaultValue={.text}
style={styles}
onChange={(value: string) => {
setValue("text", value);
}}
modules={modules}
formats={formats}
forwardedRef={quillRef}
className="text-text"
/>
Where you have printed your dynamic content, please insert this style
<div className="" style={{ whiteSpace: "pre-line" }} dangerouslySetInnerHTML={{ __html: text ?? "" }} />
Conclusion
Customizing the Enter key behavior in React Quill can enhance your rich text editing experience. This blog post shows you how to disable the default Enter behavior and add a custom binding for Shift + Enter to insert line breaks. This customization provides more control over how content is formatted in your Quill editor. Feel free to adapt and extend this solution to meet your specific requirements. Happy editing!